Can binary search be used for strings Java?
Searching a string using binary search algorithm is something tricky when compared to searching a number. Because we can compare 2 numerics directly, but in the case of strings it’s not as simple as number comparison.
Can you use binary search with strings?
Binary search is searching technique that works by finding the middle of the array for finding the element. For array of strings also the binary search algorithm will remain the same. But the comparisons that are made will be based on string comparison.
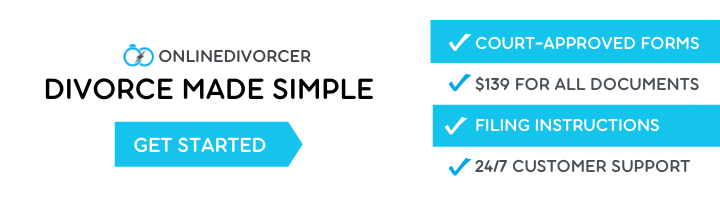
How do you find index in binary search?
If the target exists in the array, return its index. If the target does not exist, then return -1 . The binary search divides the input array by half at every step. After every step, either we find the index we are looking for, or we discard half of the array.
Does index () use binary search?
Database indexes use b-trees, a generalization of a binary tree. That’s not the same as using a binary search, although the general idea of “divide and conquer” applies in both cases.

Is database index a binary tree?
It is a Binary tree. This is the simplest form of the B-tree. For Binary tree, we can use pointers instead of keeping data in a sorted array. Mathematically, we can prove that the worst case search time for a binary tree is O(log(n)).
How does binary search work in Java?
Binary searching works by comparing an input value to the middle element of the array. The comparison determines whether the element equals the input, is less than the input, or is greater than the input.
Which condition does not belong to binary search algorithm?
Q. | Which of the following is not the required condition for binary search algorithm |
---|---|
B. | the list must be sorted |
C. | there should be the direct access to the middle element in any sublist |
D. | none of the above |
Answer» a. there must be mechanism to delete and/ or insert elements in list |
What is binary search algorithm in Java?
Binary Search in Java is a search algorithm that finds the position of a target value within a sorted array. Binary search compares the target value to the middle element of the array. It works only on a sorted set of elements. To use binary search on a collection, the collection must first be sorted.
What is binary tree index?
Representation. Binary Indexed Tree is represented as an array. Let the array be BITree[]. Each node of the Binary Indexed Tree stores the sum of some elements of the input array. The size of the Binary Indexed Tree is equal to the size of the input array, denoted as n.
What is the best way to compare strings in Java?
The right way of comparing String in Java is to either use equals(), equalsIgnoreCase(), or compareTo() method. You should use equals() method to check if two String contains exactly same characters in same order. It returns true if two String are equal or false if unequal.
How do you take input strings?
Example of next() method
- import java.util.*;
- class UserInputDemo2.
- {
- public static void main(String[] args)
- {
- Scanner sc= new Scanner(System.in); //System.in is a standard input stream.
- System.out.print(“Enter a string: “);
- String str= sc.next(); //reads string before the space.
How do you draw a binary index tree?
BIT[] is an array of size = 1 + the size of the given array a[] on which we need to perform operations. Initially all values in BIT[] are equal to 0. Then we call update() operation for each element of given array to construct the Binary Indexed Tree.
Answer: The binary search algorithm uses a divide-and-conquer strategy that repeatedly cuts the array into halves or two parts. Thus it is named as binary search. Binary search is the frequently used searching technique in Java. The requirement for a binary search to be performed is that the data should be sorted in ascending order.
How to sort array in binary search in Java?
In case of binary search, array elements must be in ascending order. If you have unsorted array, you can sort the array using Arrays.sort (arr) method. Let’s see an example of binary search in java. System.out.println (“Element is not found!”);
What is the complexity of a binary search?
If we start saving items in sorted order and search for items using the binary search, we can achieve a complexity of O (log n). With binary search, the time taken by the search results naturally increases with the size of the dataset, but not proportionately. 3. Binary Search
What is binary search&recursive binary search?
This Tutorial will Explain Binary Search & Recursive Binary Search in Java along with its Algorithm, Implementation, and Java Binary Seach Code Examples: A binary search in Java is a technique that is used to search for a targeted value or key in a collection. It is a technique that uses the “divide and conquer” technique to search for a key.