What is an iterator class in Python?
An iterator is an object that contains a countable number of values. An iterator is an object that can be iterated upon, meaning that you can traverse through all the values. Technically, in Python, an iterator is an object which implements the iterator protocol, which consist of the methods __iter__() and __next__() .
How do I make an iterator class in Python?
There are four ways to build an iterative function:
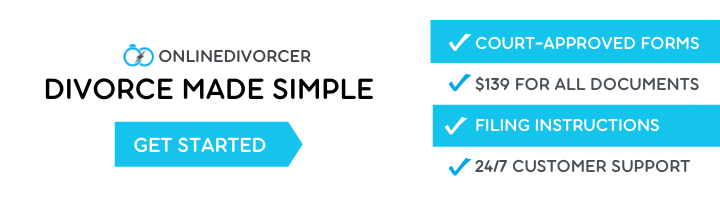
- create a generator (uses the yield keyword)
- use a generator expression (genexp)
- create an iterator (defines __iter__ and __next__ (or next in Python 2. x))
- create a class that Python can iterate over on its own (defines __getitem__ )
What is iterator in Python with example?
In Python, an iterator is an object which implements the iterator protocol, which means it consists of the methods such as __iter__() and __next__(). An iterator is an iterable object with a state so it remembers where it is during iteration. For Example, Generator.
How do you make an iterator class?
To create an Iterator class we need to override __next__() function inside our class i.e. __next__() function should be implemented in such a way that every time we call the function it should return the next element of the associated Iterable class. If there are no more elements then it should raise StopIteration.
Why are iterators useful in Python?

Iterators allow lazy evaluation, only generating the next element of an iterable object when requested. This is useful for very large data sets. Iterators and generators can only be iterated over once. Generator Functions are better than Iterators.
How do I create an iteration in Python?
You can create an iterator object by applying the iter() built-in function to an iterable. You can use an iterator to manually loop over the iterable it came from. A repeated passing of iterator to the built-in function next() returns successive items in the stream.
Why iterator is used?
Iterator in Java is used to traverse each and every element in the collection. Using it, traverse, obtain each element or you can even remove. ListIterator extends Iterator to allow bidirectional traversal of a list, and the modification of elements.
Why do we use iterator in Python?
How does iteration work in Python?
Iterator in python is an object that is used to iterate over iterable objects like lists, tuples, dicts, and sets. The iterator object is initialized using the iter() method. It uses the next() method for iteration. next ( __next__ in Python 3) The next method returns the next value for the iterable.
Is iterator a class or interface?
In Java, Iterator is an interface available in Collection framework in java. util package. It is a Java Cursor used to iterate a collection of objects. It is used to traverse a collection object elements one by one.
How do I write an iterator?
Java – How to Use Iterator?
- Obtain an iterator to the start of the collection by calling the collection’s iterator( ) method.
- Set up a loop that makes a call to hasNext( ). Have the loop iterate as long as hasNext( ) returns true.
- Within the loop, obtain each element by calling next( ).
How do you implement iterators in Python?
Technically speaking, a Python iterator object must implement two special methods, __iter__() and __next__() , collectively called the iterator protocol. An object is called iterable if we can get an iterator from it. Most built-in containers in Python like: list, tuple, string etc. are iterables.
Are generators faster than iterators?
Generators are not faster than iterators. Generators are iterators. Usually generator functions are actually slower, but more memory efficient.
How do you implement a class in Python?
A Class is like an object constructor, or a “blueprint” for creating objects.
- Create a Class. To create a class, use the keyword class :
- Create Object. Now we can use the class named MyClass to create objects:
- The self Parameter.
- Modify Object Properties.
- Delete Object Properties.
- Delete Objects.
What is an iterator class?
An Iterator is an object that can be used to loop through collections, like ArrayList and HashSet. It is called an “iterator” because “iterating” is the technical term for looping. To use an Iterator, you must import it from the java.
How can iteration be performed in Python?
Iterations are performed through ‘for’ and ‘while’ loops. Iterations execute a set of instructions repeatedly until some limiting criteria is met. In contrast to recursion, iteration does not require temporary memory to keep on the results of each iteration.
Is Python list an iterator?
A list is an iterable. But it is not an iterator. If we run the __iter__() method on our list, it will return an iterator.
How do you implement iteration in Python?
We just have to implement the __iter__() and the __next__() methods. The __iter__() method returns the iterator object itself. If required, some initialization can be performed. The __next__() method must return the next item in the sequence.
Is iterator an abstract class?
Each implementation of Iterator is unique to the collection it is iterating. These is not enough common code to warrant an abstract base class. That said the use of an interface is much less restrictive than an abstract class. Remember that in Java a class may only extend a single base class.
Why do we need iterator?
Iterator must be used whenever we want to enumerate elements in all Collection framework implemented interfaces like Set, List, Queue, Deque, and all implemented classes of Map interface. Iterator is the only cursor available for the entire collection framework.
Why do we need iterator in Python?
How to create an iterator in Python?
In python, we can create an iterator for any container object using the iter () method. The iter () method takes an iterable object as input and returns an iterator for the same object. In the output, you can see that a list_iterator object has been created by passing a list to the iter () function.
What is the difference between Python iterator and iterable?
– Iterator is an object that remembers iteration count via an internal state variable. – Nothing resets this variable back to zero when the iteration crosses the last item, instead StopIteration () is raised to indicate end of iteration. – this would mean they can only be iterated once.
Are there builtin iterators in Python?
Note that Python cannot guarantee that user-defined or 3rd party iterators implement this requirement correctly. The StopIteration exception is made visible as one of the standard exceptions. It is derived from Exception. A new built-in function is defined, iter (), which can be called in two ways: iter (obj) calls PyObject_GetIter (obj).
How to make an object iterable Python?
What is a Python iterable?