Can you multiply a list in Python?
Lists and strings have a lot in common. They are both sequences and, like pythons, they get longer as you feed them. Like a string, we can concatenate and multiply a Python list.
How do you multiply all items in a list in Python?
Use the syntax [element * number for element in list] to multiply each element in list by number .
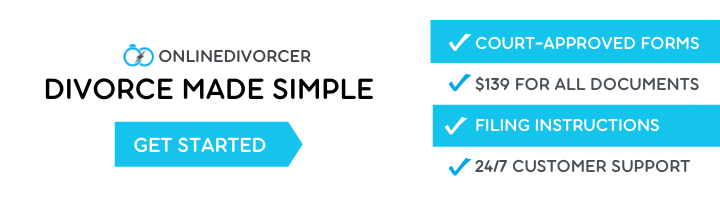
- a_list = [1, 2, 3]
- multiplied_list = [element * 2 for element in a_list]
- print(multiplied_list)
How do you multiply a list by a float in Python?
“python multiply list with float” Code Answer
- my_list = [1, 2, 3, 4, 5]
- my_new_list = [i * 5 for i in my_list]
- [5, 10, 15, 20, 25]
How do you do multiplication in Python?
In python, to multiply number, we will use the asterisk character ” * ” to multiply number. After writing the above code (how to multiply numbers in Python), Ones you will print “ number ” then the output will appear as a “ The product is: 60 ”.

How do you multiply each element in a nested list Python?
Using Loops The outer loop keeps track of number of elements in the list and the inner loop keeps track of each element inside the nested list. We use the * operator to multiply the elements of the second list with respective elements of the nested list.
How do you multiply a list in a for loop?
Use a for loop to multiply all values in a list
- a_list = [2, 3, 4]
- product = 1.
- for item in a_list: Iterate over a_list.
- product = product * item. multiply each element.
- print(product)
Can you multiply a list by a float?
Multiplying string with float You cannot multiply a string with a non – integer value. So, python will raise a TypeError saying that Python Can’t Multiply Sequence By Non-int Of Type ‘float’. It will raise the same error even if we try to multiply a list with a non – integer value.
How do you multiply two numbers in a list?
Algorithm
- Initialise the two linked lists.
- Initialise two variables with 0 to store the two numbers.
- Iterate over the two linked lists. Add each digit to the respective number variable at the end.
- Multiply the resultant numbers and store the result in a variable.
- Create a new list with the result.
- Print the new list.
How do you find the multiples of 5 in Python?
“multiple of 5 in python” Code Answer’s
- def multiples(m, count):
- for i in range(count):
- print(i*m)
How do you multiply a range in Python?
In python, to multiply number, we will use the asterisk character ” * ” to multiply number. After writing the above code (how to multiply numbers in Python), Ones you will print “ number ” then the output will appear as a “ The product is: 60 ”. Here, the asterisk character is used to multiply the number.
Can you multiply a list by a scalar?
You can use any scalar types (int, float, Boolean, etc.) and any method other than traversal with the list multiplication function in python language. The first illustration was all about using a single list; however, we have used two lists in our second illustration.
How do you multiply an array in python?
multiply() in Python. numpy. multiply() function is used when we want to compute the multiplication of two array. It returns the product of arr1 and arr2, element-wise.
How do you multiply numbers in Python?
How do you print multiplication in Python?
Example:
- number = int(input (“Enter the number of which the user wants to print the multiplication table: “))
- count = 1.
- # we are using while loop for iterating the multiplication 10 times.
- print (“The Multiplication Table of: “, number)
- while count <= 10:
- number = number * 1.
- print (number, ‘x’, i, ‘=’, number * count)
How do you find the multiples of 10 in Python?
Basically, x%y returns the value of the remainder of x/y , so if the remainder is 0 , than x is evenly divisible by y . Therefore, if x%10 returns something other than a zero, x is not divisible by 10.
How do you check for multiples of 4 in Python?
“multiple of 4 in python” Code Answer’s
- def multiples(m, count):
- for i in range(count):
- print(i*m)
How do you multiply an entire array in Python?
Numpy multiply array by scalar In order to multiply array by scalar in python, you can use np. multiply() method.
How do you multiply a range of numbers in Python?
How do you multiply a range?
- In any cell, type -1.
- Select the cell and press Ctrl+C to copy.
- Select the range to be multiplied by -1.
- Press Shift+F10 or right-click, and then select Paste.
- Special from the shortcut menu.
- Select the Multiply option button, and then click OK.
How do you multiply an array of numbers?
To find the product of elements of an array.
- create an empty variable. ( product)
- Initialize it with 1.
- In a loop traverse through each element (or get each element from user) multiply each element to product.
- Print the product.
How to perform element-wise multiplication in Python?
We can perform the element-wise multiplication in Python using the following methods: The np.multiply (x1, x2) method of the NumPy library of Python takes two matrices x1 and x2 as input, performs element-wise multiplication on input, and returns the resultant matrix as input.
How do you do elementwise multiplication with ndarrays?
With ndarrays, you can just use *for elementwise multiplication: a * b If you’re on Python 3.5+, you don’t even lose the ability to perform matrix multiplication with an operator, because @does matrix multiplication now:
What is the result of multiplication of two ndarray in Python?
Here, np.array(a)returns a 2D array of type ndarrayand multiplication of two ndarraywould result element wise multiplication. So the result would be: result = [[5, 12], [21, 32]]
How to multiply matrixobjects in Python?
4 Answers 4 ActiveOldestVotes 212 For elementwise multiplication of matrixobjects, you can use numpy.multiply: import numpy as np a = np.array([[1,2],[3,4]]) b = np.array([[5,6],[7,8]]) np.multiply(a,b)