What is bitwise or operator in Python?
Bitwise or operator: Returns 1 if either of the bit is 1 else 0. Example: a = 10 = 1010 (Binary) b = 4 = 0100 (Binary) a | b = 1010 | 0100 = 1110 = 14 (Decimal) Bitwise not operator: Returns one’s complement of the number.
What is the use of bitwise or?
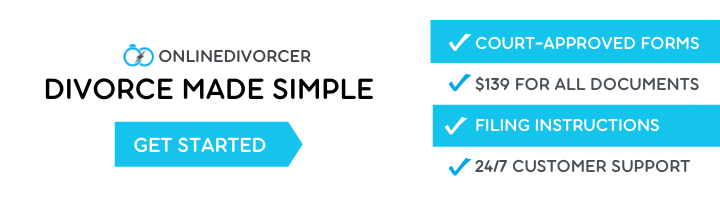
The bitwise OR operator ( | ) returns a 1 in each bit position for which the corresponding bits of either or both operands are 1 s.
What is bitwise operator in Python with example?
Overview of Python’s Bitwise Operators
Operator | Example | Meaning |
---|---|---|
^ | a ^ b | Bitwise XOR (exclusive OR) |
~ | ~a | Bitwise NOT |
<< | a << n | Bitwise left shift |
>> | a >> n | Bitwise right shift |
How do you write bitwise or operation in Python?
(~a ) = -61 (means 1100 0011 in 2’s complement form due to a signed binary number. The left operands value is moved left by the number of bits specified by the right operand. The left operands value is moved right by the number of bits specified by the right operand.

How do you write bitwise or?
The | (bitwise OR) in C or C++ takes two numbers as operands and does OR on every bit of two numbers. The result of OR is 1 if any of the two bits is 1. The ^ (bitwise XOR) in C or C++ takes two numbers as operands and does XOR on every bit of two numbers. The result of XOR is 1 if the two bits are different.
How do you do bitwise exclusive OR?
The bitwise exclusive OR operator (in EBCDIC, the ‸ symbol is represented by the ¬ symbol) compares each bit of its first operand to the corresponding bit of the second operand. If both bits are 1 ‘s or both bits are 0 ‘s, the corresponding bit of the result is set to 0 .
Is bitwise or the same as addition?
Yes, as (seen bitwise) 0+1 is the same as 0|1 . The only difference is 1|1 (=1) vs. 1+1(=0b10) , i.e. create a 0 and having overflow, affecting the bits to the left). So in your case both are equivalent.
WHAT IS and NOT or XOR in Python?
XOR in Python is also known as “exclusive or” that compares two binary numbers bitwise. If both bits are the same, the XOR operator outputs 0. If both bits are different, the XOR operator outputs 1.
What is bitwise exclusive or?
The bitwise exclusive OR operator ( ^ ) compares each bit of its first operand to the corresponding bit of its second operand. If the bit in one of the operands is 0 and the bit in the other operand is 1, the corresponding result bit is set to 1. Otherwise, the corresponding result bit is set to 0.
How do I minimize bitwise or?
The bitwise OR of all the elements of the array has to be maximized by performing one task. The task is to multiply any element of the array at-most k times with a given integer x. of the array will result the same three numbers 1, 1, 2. So, the result is 1 | 1 | 2 = 3.
What does this || mean?
The symbol for parallelism is ||. To signify that two lines are parallel, you can place the names of the lines on either side of this symbol. For example, AB || CD means that the line AB runs parallel to the line CD.
What is output of bitwise or operation?
The output of bitwise AND is 1 if the corresponding bits of two operands is 1. If either bit of an operand is 0, the result of corresponding bit is evaluated to 0.
Is the bitwise exclusive or?
What is bit exclusive or?
What is bitwise inclusive or?
The | (bitwise inclusive OR) operator compares the values (in binary format) of each operand and yields a value whose bit pattern shows which bits in either of the operands has the value 1 . If both of the bits are 0 , the result of that bit is 0 ; otherwise, the result is 1 .
How do you do bitwise or operations?
Is there a XOR operator in Python?
XOR Operator in Python is also known as “exclusive or” that compares two binary numbers bitwise if two bits are identical XOR outputs as 0 and when two bits are different then XOR outputs as 1. XOR can even be used on booleans.
How do you XOR a list in Python?
Program to find XOR sum of all pairs bitwise AND in Python
- xor1 := 0.
- xor2 := 0.
- for each a in arr1, do. xor1 := xor1 XOR a.
- for each a in arr2, do. xor2 := xor2 XOR a.
- return xor1 AND xor2.
Which symbol is represented the bitwise or?
The bitwise exclusive OR operator (in EBCDIC, the ‸ symbol is represented by the ¬ symbol) compares each bit of its first operand to the corresponding bit of the second operand.
https://www.youtube.com/watch?v=0zWiugtOMd4